Connect to Cache
Once your Montplex Cache has been created, you can start using it.
This tutorial will guide you in detail on how to connect and use Montplex Cache.
Cache Details
After the cache cluster is successfully created, you can view the current cache cluster's details on the Cache details page. This includes connection information such as Endpoint, Password, and Port, as well as the product group to which the cache belongs, current cluster information, the number of shards, and memory usage.
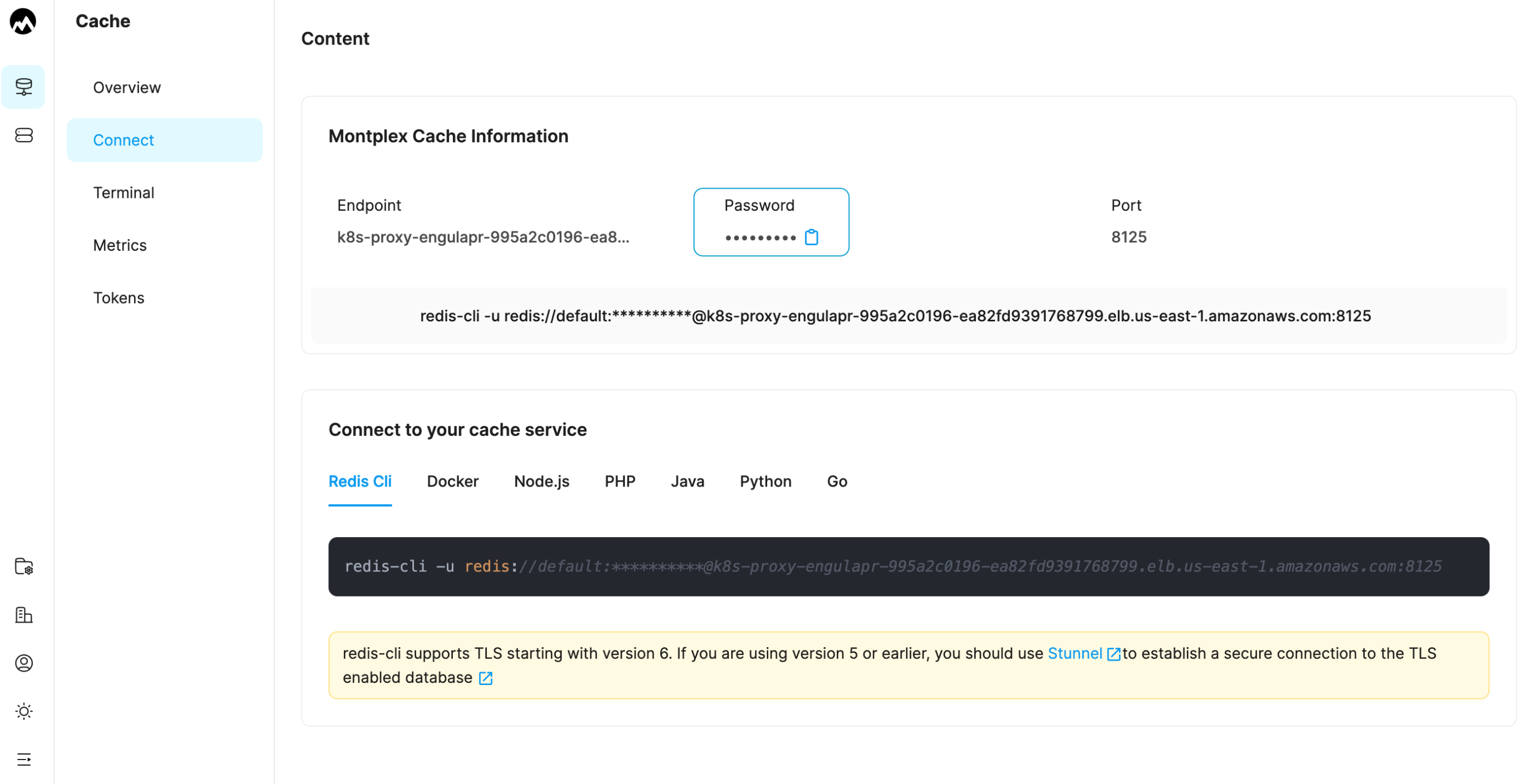
Examples
Redis Cli
1redis-cli -h YOUR_ENDPOINT -p YOUR_PORT -a YOUR_PASSWORD
Node.js
Library ioredis
1const Redis = require('ioredis')
2
3const client = new Redis({
4 port: 'YOUR_PORT',
5 host: 'YOUR_ENDPOINT',
6 password: 'YOUR_PASSWORD'
7})
8
9client.set('foo', 'bar')
Library node-redis
1const redis = require('redis')
2const client = redis.createClient({
3 url: 'YOUR_ENDPOINT',
4 port: 'YOUR_PORT',
5 password: 'YOUR_PASSWORD',
6})
7
8client.on('error', (err) => {
9 throw err
10})
11
12client.set('foo', 'bar')
PHP
Library predis
1$client = new PredisClient(
2 [
3 'host' => 'YOUR_ENDPOINT',
4 'password' => 'YOUR_PASSWORD',
5 'port' => 'YOUR_PORT',
6 'scheme' => tcp,
7 ]
8);
9$client->set("foo", "bar");
10print_r($client->get("foo"));
Library phpredis
1$redis = new Redis();
2$redis->connect('YOUR_ENDPOINT', YOUR_PORT);
3$redis->auth('YOUR_PASSWORD');
4
5$redis->set("foo", "bar");
6print_r($redis->get("foo"));
Python
Library redis-py
1import redis
2r = redis.Redis(
3 host= 'YOUR_ENDPOINT',
4 port= 'YOUR_PORT',
5 password= "YOUR_PASSWORD"
6)
7
8r.set('foo','bar')
9print(r.get('foo'))
Java
Library jedis
1public static void main(String[] args) {
2 Jedis jedis = new Jedis("YOUR_ENDPOINT", "YOUR_PORT");
3 jedis.auth("YOUR_PASSWORD");
4 jedis.set("foo", "bar");
5 String value = jedis.get("foo");
6}
Go
Library go-redis
1var ctx = context.Background()
2
3func main() {
4
5 opt := redis.NewClient(&redis.Options{
6 Addr: "YOUR_ENDPOINT:YOUR_PORT",
7 Password: "YOUR_PASSWORD"
8 })
9
10 client := redis.NewClient(opt)
11
12 client.Set(ctx, "foo", "bar", 0)
13 val := client.Get(ctx, "foo").Val()
14 print(val)
15
16}
Library: redigo
1func main() {
2
3 c, err := redis.Dial("tcp", "YOUR_ENDPOINT:YOUR_PORT")
4 if err != nil { panic(err) }
5
6 _, err = c.Do("AUTH", "YOUR_PASSWORD")
7 if err != nil { panic(err) }
8
9 _, err = c.Do("SET", "foo", "bar")
10 if err != nil { panic(err) }
11
12 value, err := redis.String(c.Do("GET", "foo"))
13 if err != nil { panic(err) }
14
15 println(value)
16}
Docker
1docker run -it redis:alpine redis-cli -h YOUR_ENDPOINT -p YOUR_PORT -a YOUR_PASSWORD